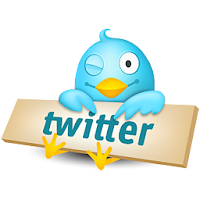
Just follow the simple steps below using PERL. Hope you like it!
1. Install LWP::UserAgent in your server.
2. Initialize libraries, header and user agent.
require LWP::UserAgent;
my $lwpua = LWP::UserAgent->new;
my $uagent = "Mozilla/5.0 (Windows; U; Windows NT 5.1; en-US; rv:1.8.0.6) Gecko/20060728 Firefox/1.5.0.6";
my @header = ('Referer' => 'http://api.twitter.com/',
'User-Agent' => $uagent);
3. Compose the HTTP request.
my $twuser = '<your twitter username>';
my $twurl = "http://api.twitter.com/1/statuses/user_timeline.<format>?screen_name=$twuser";
Supported Format:
- JSON
- XML
- RSS
- ATOM
- user_id - The id of twitter account you want to retrieve.
- screen_name - The username of twitter account you want to retrieve.
- since_id - This will return the feeds after or more than the specified message id.
- max_id - This will return the feeds before or less than the specified message id.
- count - The number of tweets to be retrieve.
- page - This will specify the page of the result to retrieve.
- trim_user - When set to either true, t or 1, each tweet returned in a timeline will include a user object including only the status authors numerical id.
- include_rts - When set to either true, t or 1, the timeline will contain native retweets (if they exist) in addition to the standard stream of tweets.
- include_entities - When set to either true, t or 1, each tweet will include a node called "entities". This node offers a variety of metadata about the tweet in a discreet structure, including: user_mentions, urls, and hashtags. While entities are opt-in on timelines at present, they will be made a default component of output in the future.
my $response = $lwpua->get($twurl, @header);
my $return = $response->content;
That's it!! Please see below for the complete code using JSON format.
#!/usr/bin/perl
require LWP::UserAgent;
use JSON;
my $lwpua = LWP::UserAgent->new;
my $uagent = "Mozilla/5.0 (Windows; U; Windows NT 5.1; en-US; rv:1.8.0.6) Gecko/20060728 Firefox/1.5.0.6";
my @header = ('Referer' => 'http://api.twitter.com/', 'User-Agent' => $uagent);
my $twuser = '<your twitter username>';
my $twurl = "http://api.twitter.com/1/statuses/user_timeline.json?screen_name=$twuser";
my $response = $lwpua->get($twurl, @header);
my $return = $response->content;
my $json = JSON->new->allow_nonref;
my $json_text = $json->decode($return);
my @tweets = @{$json_text};
my $message;
foreach $tweet (@tweets)
{
$message .= $tweet->{text}."\n";
}
print "$message";
1;
Resource: dev.twitter.com
No comments:
Post a Comment