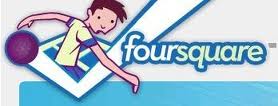
Foursquare follows authentication via OAuth and this post will teach you how to do it via back-end using PERL and PHP.
I assume you already read my post on how to Login to foursquare via back-end in PERL. You should be able to understand it first to proceed with authentication and do the check-in in users behalf.
Just follow the simple steps for your implementation.
1. You must have a Client ID and the Callback URL. If you don't have yet, create one by going to foursquare developers link - http://developer.foursquare.com/
2. To register a new consumer, click the "Manage OAuthConsumers" link or go to OAuth page - https://foursquare.com/oauth/
3. Enter your Application Name, Application Website, and Callback URL. Please take note that your Callback URL will be your Redirect URI.
4. After successful registration, Client ID and Client Secret will be generated.
5. What we need from now is the Client ID and once we have it, we can now start the coding part.
6. First thing we have to do is to login to users account via back-end. Please see post - Login to foursquare via back-end in PERL
7. After successful login, next step is to authenticate your application. To authenticate your app, you should pass a Client ID and your Callback URL that was registered from Consumer Registration.
$client_id = '<your client id>';
$redirect_uri = '<your callback url>';
$response = $lwpua->get("https://foursquare.com/oauth2/authenticate?client_id=$client_id&response_type=code&display=touch&redirect_uri=$redirect_uri", @header);
$form_data = $response->content;
$form_data =~ s/\n//g;
8. If this is the first time you authenticate your app, return page will be asking users permission to "Allow" the application to access account details and transact in users behalf. We can do this in back-end by implementing REGEX to get the NAME parameter of the value="Allow", then passed it on to authentication URL - https://foursquare.com/oauth2/authenticate.
$form_data =~ /form method="post" action="(.*?)"(.*?)input value="Allow" type="submit" name="(.*?)" class="input_button"/ig;
$form_action = $1;
$form_allow = $3;
$response = $lwpua->get("https://foursquare.com/oauth2/authenticate?$form_allow=Allow", @header);
$form_data = $response->content;
9. After allowing the application, foursquare will redirect the user to redirect URI that we set on step #7. Your callback URL or redirect URI must capture the CODE parameter and exchange it with TOKEN. This can now be done via PHP.
10. Foursquare will pass the CODE parameter via GET method on our Callback URL. Our Callback URL should be able to capture it and use it in exchange of an access token. To get an access token, we should pass the CODE parameter, Client ID, Client Secret, Callback URL, and Grant Type to the access token URL - https://foursquare.com/oauth2/access_token. Client ID, Client Secret, and Callback URL are data from the consumer registration, and the Grant Type should be equal to "authorization_code". Please take note that this is now on PHP.
$client_id = '<your client id>';
$client_secret = '<your client secret>';
$redirect_uri = '<your callback url>';
$grant_type = 'authorization_code';
$code = $_GET['code'];
$oauthurl = "https://foursquare.com/oauth2/access_token?client_id=$client_id&client_secret=$client_secret&grant_type=$grant_type&redirect_uri=$redirect_uri&code=$code";
11. As per implementation below, I use the open stream to get the contents and convert it to JSON which will then return an object with an access token.
$url_handler = fopen("$oauthurl", 'r');
$json = json_decode(stream_get_contents($url_handler));
fclose($url_handler);
$token = $json->access_token;
13. Now that you have the Token, we are now ready to do the Foursquare Check-in. Going back to PERL, what we need to do here is to do a POST request to Foursquare API URL - https://api.foursquare.com/v2/checkins/add. venueId will be the place or location you want to check-in, shout is optional if you want your post to have a custom message, broadcast can be of public or private, and lastly the oauth_token which is the access token we get from step #12. Again, this is now in PERL.
$intVenueID = "<the venue id you want to check-in>";
$strShout = "<your shout message>";
$strToken = "<your access token>";
$response = $lwpua->post("https://api.foursquare.com/v2/checkins/add",
['venueId' => $intVenueID,
'shout' => $strShout,
'oauth_token' => $strToken,
'broadcast' => 'public,faceboook,twitter'], @header);
print $response->content;
That's it!! Hope you were able to follow. In case you don't, you can refer to the scripts below. Good luck and enjoy!!
- Here's the PERL script, it composes of 2 sub functions: check_foursquare_login() and post_foursquare_checkin()
#!/usr/bin/perl
require LWP::UserAgent;
use strict;
use warnings;
use HTTP::Cookies;
my $lwpua = LWP::UserAgent->new;
my $user_agent = "Mozilla/5.0 (Windows; U; Windows NT 5.1; en-US; rv:1.8.0.6) Gecko/20060728 Firefox/1.5.0.6";
my @header = ('Referer' => 'https://foursquare.com',
'User-Agent' => $user_agent);
my $cookie_file = "cookies.dat";
my $cookie_jar = HTTP::Cookies->new(
file => $cookie_file,
autosave => 1,
ignore_discard => 1);
$lwpua->cookie_jar($cookie_jar);
my $strUser = "<your foursquare username>";
my $strPass = "<your foursquare password>";
my $client_id = "15NGPG2GHI3S1UFMST01FOO0ABPXNFFTGJVTVSZS55W1UN4A";
my $redirect_uri = "http://localhost/foursquare/foursquare.php";
my $status = "testing foursquare status";
my $form_data = &check_foursquare_login($strUser, $strPass, $client_id, $redirect_uri);
if ($form_data =~ /^OK\|/)
{
my ($strTag, $intUserID, $strToken) = split /\|/, $form_data, 3;
&post_foursquare_checkin($intUserID, $strToken, '', $status);
unlink($cookie_file);
print "done!";
}
else
{
$form_data =~ /form method="post" action="(.*?)"(.*?)input value="Allow" type="submit" name="(.*?)" class="input_button"/ig;
my $form_action = $1;
my $form_allow = $3;
my $response = $lwpua->get("https://foursquare.com/oauth2/authenticate?$form_allow=Allow", @header);
$form_data = $response->content;
if ($form_data =~ /^OK\|/)
{
my ($strTag, $intUserID, $strToken) = split /\|/, $form_data, 3;
&post_foursquare_checkin($intUserID, $strToken, '', $status);
unlink($cookie_file);
print "done!";
}
else
{
unlink($cookie_file);
print "error authentication!";
}
}
sub check_foursquare_login
{
my ($strUser, $strPass, $client_id, $redirect_uri) = @_;
# log-in to foursquare
my $response = $lwpua->post('https://foursquare.com/mobile/login',
['username' => $strUser,
'password' => $strPass], @header);
$cookie_jar->extract_cookies( $response );
$cookie_jar->save;
$response = $lwpua->get("https://foursquare.com/oauth2/authenticate?client_id=$client_id&response_type=code&display=wap&redirect_uri=$redirect_uri", @header);
$form_data = $response->content;
$form_data =~ s/\n//g;
return $form_data;
}
sub post_foursquare_checkin
{
my ($strUserID, $strToken, $intVenueID, $strShout) = @_;
my ($response);
$response = $lwpua->post("https://api.foursquare.com/v2/checkins/add",
['venueId' => $intVenueID,
'shout' => $strShout,
'oauth_token' => $strToken,
'broadcast' => 'public,faceboook,twitter'], @header);
return $response->content;
}
1;
- Here's the PHP script. Save this in your web server and set this as your callback URL in consumer registration.
<?
$client_id = "15NGPG2GHI3S1UFMST01FOO0ABPXNFFTGJVTVSZS55W1UN4A"; // hotshots client id
$client_secret = "NEHCL3UL0KS1QJD22NLIQ5RMR4BL4IUZIJZW25VHSJ4JPZKB";
$grant_type = "authorization_code";
$redirect_uri = "http://localhost/foursquare/foursquare.php";
$code = $_GET['code'];
if ($code) {
// get access token
$oauthurl = "https://foursquare.com/oauth2/access_token?client_id=$client_id&client_secret=$client_secret&grant_type=$grant_type&redirect_uri=$redirect_uri&code=$code";
$url_handler = fopen("$oauthurl", 'r');
$json = json_decode(stream_get_contents($url_handler));
fclose($url_handler);
$token = $json->access_token;
if ($token) {
$info_contents = `curl https://api.foursquare.com/v2/users/self?oauth_token=$token`;
$json = json_decode($info_contents);
$userid = $json->response->user->id;
echo "OK|$userid|$token";
}
}
?>
IMHO it would be easier to use some oAuth module from CPAN.
ReplyDeleteThis is a nice article..
ReplyDeleteIts very easy to understand ..
And this article is using to learn something about it..
web designing/development
Thanks a lot..!
yes chorny, you can use oauth module from cpan, but this article will teach you the basic.
ReplyDeletethanks muhammad azeem.. appreciate a lot..
ReplyDeleteNice post, Paul! Very useful indeed
ReplyDeleteThanks Eric for the comment.
ReplyDeleteHi,
ReplyDeleteI executed your script .I got this error.could you help me?
ERROR is:
"Use of uninitialized value $form_allow in concatenation (.) or string at C:\Docu
ments and Settings\mob100001629\Desktop\elance\foresquare\foursquare.pl line 49.
error authentication!
Make sure you supply the correct username, password, client id, and redirect uri.. can you send me a snapshot of your script? i want to see how you do it.. thanks.
ReplyDeleteThis is my script..
ReplyDeleteI got the content after login page.but I got error like this
"Use of uninitialized value $form_allow in concatenation (.) or string at C:\Docu
ments and Settings\mob100001629\Desktop\elance\foresquare\foursquare.pl line 49.
error authentication!.."
could you help me ?
require LWP::UserAgent;use strict;use warnings;use HTTP::Cookies;my $lwpua = LWP::UserAgent->new;
my $user_agent = "Mozilla/5.0 (Windows; U; Windows NT 5.1; en-US; rv:1.8.0.6) Gecko/20060728 Firefox/1.5.0.6";
my @header = ('Referer' => 'http://foursquare.com','User-Agent' => $user_agent);
my $cookie_file = "cookies.dat";
my $cookie_jar = HTTP::Cookies->new( file => $cookie_file, autosave => 1, ignore_discard => 1);
$lwpua->cookie_jar($cookie_jar);
my $strUser = "fnamelname666\@gmail.com";
my $strPass = "Team\@123";
my $client_id = "5SPMBUN1AZAG30TNAXI51LHT3XTYEFWQ54DL4OUJ42LAFYRF";
my $redirect_uri = "https://foursquare.com/signup/\?source=login_page";
my $form_data = &check_foursquare_login($strUser, $strPass, $client_id, $redirect_uri);
my ($strTag, $strToken,$status) ;
if ($form_data =~m/^OK\|/is){
($strTag, $strToken) = split /\|/, $form_data, 2;
&post_foursquare_checkin($strToken, $status);
unlink($cookie_file);
print "done!";}
else{
$form_data =~/form method="post" action="(.*?)"(.*?)input value="Allow" type="submit" name="(.*?)" class="input_button"/igs;
my $form_action = $1;
my $form_allow = $3;
my $response = $lwpua->get("https://foursquare.com/oauth2/authenticate?$form_allow=Allow", @header);
my $form_data = $response->content;
if ($form_data =~/^OK\|/){
($strTag, $strToken) = split /\|/, $form_data, 2;
&post_foursquare_checkin($strToken, $status);
unlink($cookie_file);
print "done!";
}
else{
unlink($cookie_file);
print "error authentication!";
}
}
sub check_foursquare_login{
my ($strUser, $strPass, $client_id, $redirect_uri) = @_;
# log-in to foursquare
my $response = $lwpua->post('https://foursquare.com/mobile/login', ['username' => $strUser, 'password' => $strPass], @header);
$cookie_jar->extract_cookies( $response );
$cookie_jar->save;
$response = $lwpua->get("https://foursquare.com/oauth2/authenticate?client_id=$client_id&response_type=code&display=touch&redirect_uri=$redirect_uri", @header);
my $form_data = $response->content;
$form_data =~ s/\n//g;
return $form_data;
}
sub post_foursquare_checkin{
my ($strToken, $intVenueID, $strShout) = @_;
my $response = $lwpua->post("https://api.foursquare.com/v2/checkins/add",['venueId' => $intVenueID, 'shout' => $strShout,'oauth_token' => $strToken,'broadcast' => 'public,faceboook,twitter'], @header);
return $response->content;
}
1;
hi,
ReplyDeletei did not use your php script .i executed only my perl script.may i know why php script is needed
Below i mentioned user name and password and client id and callbackurl
my $strUser = "fnamelname666\@gmail.com";
my $strPass = "Team\@123";
my $client_id = "5SPMBUN1AZAG30TNAXI51LHT3XTYEFWQ54DL4OUJ42LAFYRF";
my $redirect_uri = "https://foursquare.com/signup/\?source=login_page";
could you help me ?
This is my script
ReplyDeleterequire LWP::UserAgent;use strict;use warnings;use HTTP::Cookies;my $lwpua = LWP::UserAgent->new;
my $user_agent = "Mozilla/5.0 (Windows; U; Windows NT 5.1; en-US; rv:1.8.0.6) Gecko/20060728 Firefox/1.5.0.6";
my @header = ('Referer' => 'http://foursquare.com','User-Agent' => $user_agent);
my $cookie_file = "cookies.dat";
my $cookie_jar = HTTP::Cookies->new( file => $cookie_file, autosave => 1, ignore_discard => 1);
$lwpua->cookie_jar($cookie_jar);
my $strUser = "fnamelname666\@gmail.com";
my $strPass = "Team\@123";
my $client_id = "5SPMBUN1AZAG30TNAXI51LHT3XTYEFWQ54DL4OUJ42LAFYRF";
my $redirect_uri = "https://foursquare.com/signup/\?source=login_page";
my $form_data = &check_foursquare_login($strUser, $strPass, $client_id, $redirect_uri);
my ($strTag, $strToken,$status) ;
if ($form_data =~m/^OK\|/is){
($strTag, $strToken) = split /\|/, $form_data, 2;
&post_foursquare_checkin($strToken, $status);
unlink($cookie_file);
print "done!";}
else{
$form_data =~/form method="post" action="(.*?)"(.*?)input value="Allow" type="submit" name="(.*?)" class="input_button"/igs;
my $form_action = $1;
my $form_allow = $3;
my $response = $lwpua->get("https://foursquare.com/oauth2/authenticate?$form_allow=Allow", @header);
my $form_data = $response->content;
if ($form_data =~/^OK\|/){
($strTag, $strToken) = split /\|/, $form_data, 2;
&post_foursquare_checkin($strToken, $status);
unlink($cookie_file);
print "done!";
}
else{
unlink($cookie_file);
print "error authentication!";
}
}
sub check_foursquare_login{
my ($strUser, $strPass, $client_id, $redirect_uri) = @_;
# log-in to foursquare
my $response = $lwpua->post('https://foursquare.com/mobile/login', ['username' => $strUser, 'password' => $strPass], @header);
$cookie_jar->extract_cookies( $response );
$cookie_jar->save;
$response = $lwpua->get("https://foursquare.com/oauth2/authenticate?client_id=$client_id&response_type=code&display=touch&redirect_uri=$redirect_uri", @header);
my $form_data = $response->content;
$form_data =~ s/\n//g;
return $form_data;
}
sub post_foursquare_checkin{
my ($strToken, $intVenueID, $strShout) = @_;
my $response = $lwpua->post("https://api.foursquare.com/v2/checkins/add",['venueId' => $intVenueID, 'shout' => $strShout,'oauth_token' => $strToken,'broadcast' => 'public,faceboook,twitter'], @header);
return $response->content;
}
1;
this is my script
ReplyDeletecould you help me?
require LWP::UserAgent;use strict;use warnings;use HTTP::Cookies;my $lwpua = LWP::UserAgent->new;
my $user_agent = "Mozilla/5.0 (Windows; U; Windows NT 5.1; en-US; rv:1.8.0.6) Gecko/20060728 Firefox/1.5.0.6";
my @header = ('Referer' => 'http://foursquare.com','User-Agent' => $user_agent);
my $cookie_file = "cookies.dat";
my $cookie_jar = HTTP::Cookies->new( file => $cookie_file, autosave => 1, ignore_discard => 1);
$lwpua->cookie_jar($cookie_jar);
my $strUser = "fnamelname666\@gmail.com";
my $strPass = "Team\@123";
my $client_id = "5SPMBUN1AZAG30TNAXI51LHT3XTYEFWQ54DL4OUJ42LAFYRF";
my $redirect_uri = "https://foursquare.com/signup/\?source=login_page";
my $form_data = &check_foursquare_login($strUser, $strPass, $client_id, $redirect_uri);
my ($strTag, $strToken,$status) ;
if ($form_data =~m/^OK\|/is){
($strTag, $strToken) = split /\|/, $form_data, 2;
&post_foursquare_checkin($strToken, $status);
unlink($cookie_file);
print "done!";}
else{
$form_data =~/form method="post" action="(.*?)"(.*?)input value="Allow" type="submit" name="(.*?)" class="input_button"/igs;
my $form_action = $1;
my $form_allow = $3;
my $response = $lwpua->get("https://foursquare.com/oauth2/authenticate?$form_allow=Allow", @header);
my $form_data = $response->content;
if ($form_data =~/^OK\|/){
($strTag, $strToken) = split /\|/, $form_data, 2;
&post_foursquare_checkin($strToken, $status);
unlink($cookie_file);
print "done!";
}
else{
unlink($cookie_file);
print "error authentication!";
}
}
sub check_foursquare_login{
my ($strUser, $strPass, $client_id, $redirect_uri) = @_;
# log-in to foursquare
my $response = $lwpua->post('https://foursquare.com/mobile/login', ['username' => $strUser, 'password' => $strPass], @header);
$cookie_jar->extract_cookies( $response );
$cookie_jar->save;
$response = $lwpua->get("https://foursquare.com/oauth2/authenticate?client_id=$client_id&response_type=code&display=touch&redirect_uri=$redirect_uri", @header);
my $form_data = $response->content;
$form_data =~ s/\n//g;
return $form_data;
}
sub post_foursquare_checkin{
my ($strToken, $intVenueID, $strShout) = @_;
my $response = $lwpua->post("https://api.foursquare.com/v2/checkins/add",['venueId' => $intVenueID, 'shout' => $strShout,'oauth_token' => $strToken,'broadcast' => 'public,faceboook,twitter'], @header);
return $response->content;
}
1;
hi,
ReplyDeletei posted more comments in this blog. but It is not visible in this this blog.May i know the reason?
hi Hotshots,
ReplyDeleteI need your help.As you said where to send my script.I posted my script in this blog, but it is not visible in this blog
send it to my gmail account paulgonzaga80@gmail.com
ReplyDeleteor you can just send me your client id and redirect uri..
ReplyDeletehi,
ReplyDeletethis is my client id-5SPMBUN1AZAG30TNAXI51LHT3XTYEFWQ54DL4OUJ42LAFYRF
and redirect uri-https://foursquare.com/signup/?source=login_page.
sorry i dont know if my redirect uri correct or not.help in this.
hi Hotshots,
ReplyDeleteI need to scrape data from foursquare.com. They currently have an api (https://developer.foursquare.com/docs/) that I think the data can come from. I am looking to scrape all their data for locations.
do you have any idea about this?
hi,
ReplyDeleteIf people search possible by foursquare API
hi senthil, to help you with.. I just modified the sample script to have a client id and redirect uri in place.. you should also have a php script to be able to checkin via backend. i'll send you the script as well on your email. goodluck!! ask me again for anything..
ReplyDeleteabout the locations.. i believe you can also do this via foursquare api.. not been explore yet.. but maybe if i have time.. i will.. i'll post it to my blog once i got it.
ReplyDeletehi Paul Gonzaga ,
ReplyDeleteThank you for your reply.My requirements is that if i search people name in foursquare api search box
I will get list of people name.i need to do that via foursquare api.I am waiting for your reply
Hi Senthil,
ReplyDeleteTry this function and supply the oauth_token and search keyword.
sub search_foursquare_user
{
my ($strToken, $strUser) = @_;
my ($response);
$response = $lwpua->post("https://api.foursquare.com/v2/users/search",
['name' => $strUser,
'oauth_token' => $strToken], @header);
return $response->content;
}
Hi Hotshots,
ReplyDeleteThis code works for me..Thank you so much...Keep it up this good work thank you
no worries.. thank you for reading this blog..
ReplyDeleteThis script doesn't accept the GPS coordinates of the checkin?
ReplyDeleteI'm trying to build a proper 4 square app and looking for direction. Proper checkin with GPS cordinates... Any idea?
i believed GPS coordinates can be passed on. you only need longtitude and latitude.
ReplyDeletePlease try function below that I just created.
sub post_foursquare_checkin_longlat
{
my ($strUserID, $strToken, $intLat, $intLong, $strShout) = @_;
my ($response);
$response = $lwpua->post("https://api.foursquare.com/v2/checkins/add",
['ll' => "$intLat,$intLong",
'shout' => $strShout,
'oauth_token' => $strToken,
'broadcast' => 'public,faceboook,twitter'], @header);
return $response->content;
}
Please take note of the parameter "ll" which is corresponds to Latitude and Longtitude.
ReplyDelete